在工程属性-->链接器-->添加以下库
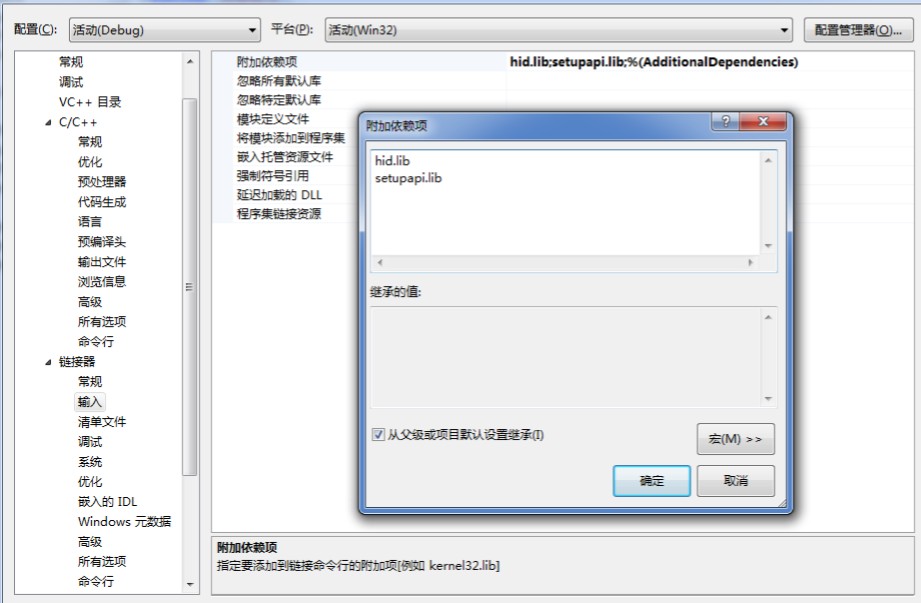
open 打开,close 关闭,打开后将获得reader 与writer 的handle,分别进行读写即可
#pragma once
#ifdef __cplusplus
extern "C" {
#endif
// This file is in the Windows DDK available from Microsoft.
#include "hidsdi.h"
#include <setupapi.h>
#include <dbt.h>
#include <setupapi.h>
int myUsbDeviceOpen(HANDLE *handle,HANDLE *hReader,HANDLE *hWriter, WORD wVID, WORD wPID);
void myUsbDeviceClose(HANDLE *handle,HANDLE *hreader,HANDLE *writer);
int myUsbDeviceWrite(HANDLE handle, LPCVOID lpBuffer, DWORD dwSize);
int myUsbDeviceRead(HANDLE handle, LPVOID lpBuffer, DWORD dwSize);
#ifdef __cplusplus
}
#endif
实现代码
#include "stdafx.h"
#include "myUSB_HID.h"
#ifdef __cplusplus
extern "C" {
#endif
HIDP_CAPS Capabilities;
PSP_INTERFACE_DEVICE_DETAIL_DATA HidFunctionClassData = NULL;
int myUsbDeviceOpen(HANDLE *handle,HANDLE *hReader,HANDLE *hWriter, WORD wVID, WORD wPID)
{
HANDLE hd;
HANDLE reader;
HANDLE writer;
BOOL bRet = FALSE;
GUID hidGuid;
HDEVINFO hardwareDeviceInfo;
SP_INTERFACE_DEVICE_DATA deviceInfoData;
ULONG predictedLength = 0;
ULONG requiredLength = 0;
PHIDP_PREPARSED_DATA PreparsedData;
hd = *handle;
*handle = NULL;
CloseHandle(hd);
hd = INVALID_HANDLE_VALUE;
deviceInfoData.cbSize = sizeof(SP_INTERFACE_DEVICE_DATA);
HidD_GetHidGuid(&hidGuid);
hardwareDeviceInfo = SetupDiGetClassDevs(&hidGuid, NULL, NULL, (DIGCF_PRESENT|DIGCF_DEVICEINTERFACE));
for (int i=0; i<128; i++)
{
if (!SetupDiEnumDeviceInterfaces(hardwareDeviceInfo, 0, &hidGuid, i, &deviceInfoData))
continue;
SetupDiGetDeviceInterfaceDetail(hardwareDeviceInfo, &deviceInfoData, NULL, 0, &requiredLength, NULL);
predictedLength = requiredLength;
HidFunctionClassData = (PSP_INTERFACE_DEVICE_DETAIL_DATA)malloc(predictedLength);
if (!HidFunctionClassData)
continue;
HidFunctionClassData->cbSize = sizeof(SP_INTERFACE_DEVICE_DETAIL_DATA);
requiredLength = 0;
if (!SetupDiGetDeviceInterfaceDetail (hardwareDeviceInfo, &deviceInfoData, HidFunctionClassData, predictedLength, &requiredLength, NULL))
continue;
hd = CreateFile (HidFunctionClassData->DevicePath, 0, FILE_SHARE_READ|FILE_SHARE_WRITE, (LPSECURITY_ATTRIBUTES)NULL,OPEN_EXISTING, 0, NULL);//FILE_SHARE_READ|FILE_SHARE_WRITE
if (hd != INVALID_HANDLE_VALUE)
{
HIDD_ATTRIBUTES attri;
HidD_GetAttributes(hd, &attri);
if ((attri.VendorID == wVID) &&
(attri.ProductID == wPID))
{
writer=CreateFile (HidFunctionClassData->DevicePath, GENERIC_WRITE, FILE_SHARE_READ|FILE_SHARE_WRITE, (LPSECURITY_ATTRIBUTES)NULL,OPEN_EXISTING, 0, NULL);
reader=CreateFile(HidFunctionClassData->DevicePath,GENERIC_READ,FILE_SHARE_READ|FILE_SHARE_WRITE,(LPSECURITY_ATTRIBUTES)NULL,OPEN_EXISTING,0,NULL);
HidD_GetPreparsedData(hd,&PreparsedData);
HidP_GetCaps(PreparsedData,&Capabilities);
bRet = TRUE;
break;
}
CloseHandle(hd);
hd = INVALID_HANDLE_VALUE;
}
free(HidFunctionClassData);
}
SetupDiDestroyDeviceInfoList(hardwareDeviceInfo);
*handle= hd;
*hReader= reader;
*hWriter = writer;
return bRet;
}
void myUsbDeviceClose(HANDLE *handle,HANDLE *hreader,HANDLE *writer)
{
CloseHandle(*hreader);
*hreader = INVALID_HANDLE_VALUE;
CloseHandle(*writer);
*writer = INVALID_HANDLE_VALUE;
CloseHandle(*handle);
*handle = INVALID_HANDLE_VALUE;
if(HidFunctionClassData!=NULL)
{
free(HidFunctionClassData);
HidFunctionClassData = NULL;
}
}
int myUsbDeviceWrite(HANDLE handle, LPCVOID lpBuffer, DWORD dwSize)
{
BYTE wBuffer[1024] = {0};
DWORD dwRet = 0;
BOOL bRet;
wBuffer[0] = 0x00;
wBuffer[1] = (unsigned char)dwSize;
memcpy(&wBuffer[2], lpBuffer, min(Capabilities.OutputReportByteLength, dwSize));
bRet = WriteFile(handle, wBuffer, Capabilities.OutputReportByteLength, &dwRet, NULL);
return bRet;
}
int myUsbDeviceRead(HANDLE handle, LPVOID lpBuffer, DWORD dwSize)
{
BYTE rBuffer[1024] = {0};
DWORD dwRet;
BOOL bRet;
rBuffer[0] = 0x00;
bRet = ReadFile(handle, rBuffer, Capabilities.InputReportByteLength, &dwRet, NULL);
memcpy(lpBuffer, &rBuffer[1], min(rBuffer[0], dwSize));
return bRet;
}
#ifdef __cplusplus
}
#endif